Python Interview Questions
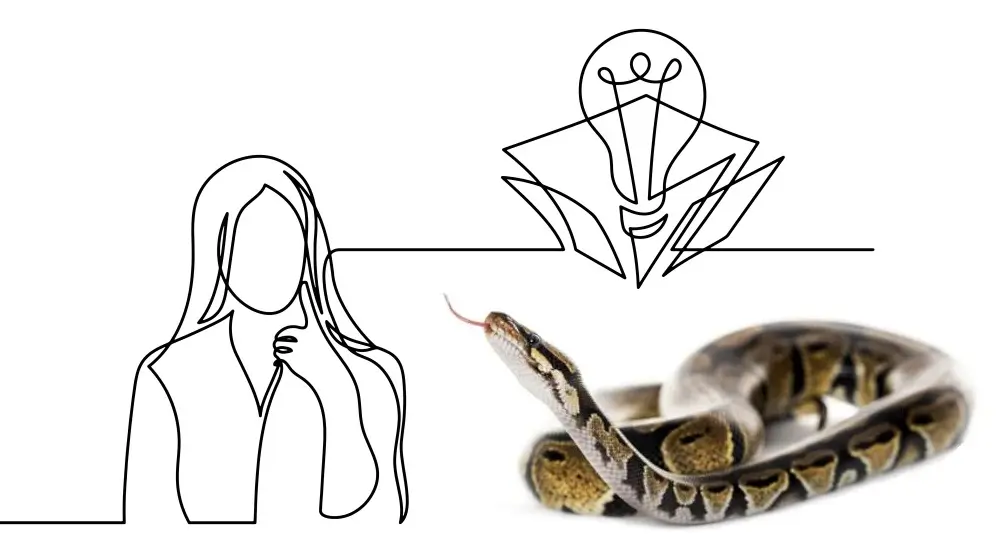
Here is a list of common Python interview questions with detailed answers to help you prepare for the interview as a Python developer.
Python, with its versatile use cases and straightforward syntax, has seen its popularity growing continuously in software development, data science, artificial intelligence, and many other fields.
As such, interviews for Python-related positions are designed not only to test a candidate’s knowledge of the language’s syntax but also to assess their problem-solving abilities, understanding of efficient coding practices, and familiarity with Python’s vast ecosystem of libraries and frameworks.
Whether you’re a fresher looking to land your first job as a Python developer or a seasoned developer aiming to make a leap into a Python-centric role, being well-prepared for your interview is crucial. This list of Python interview questions and answers aims to assist you with brushing up on Python programming concepts to prepare for your interview.
The questions listed here range from fundamental concepts to advanced programming techniques. These questions have been carefully selected to cover a broad spectrum of Python’s features and libraries, ensuring you can approach your interview with confidence.
We’ve got you covered, from exploring Python’s dynamic typing system and its approach to object-oriented programming to diving into more complex topics like concurrency, decorators, and the Global Interpreter Lock (GIL).
So, whether you’re preparing for a technical interview or just looking to brush up on your Python skills, this article is your go-to resource for the most relevant and challenging Python interview questions. Let’s dive in and explore what it takes to excel in your Python programming interview.
Python Programming Questions and Answers
Q1. What are the key features of Python?
Some of the key features of Python are
- Dynamic Typing: Python does not require declaring the type of variables; the interpreter infers the type at runtime.
- Garbage Collection: Automatic memory management to free up unused memory.
- Standard Library: A large library of modules and functions for rapid development.
- Support for Multiple Paradigms: Including procedural, object-oriented, and functional programming.
- Extensibility: Allows integration with other languages like C or C++ for performance-critical components.
- Portability: Runs on various operating systems without modification.
- Interpreted Nature: Executes code line by line, which makes debugging easier.
- Readability: Emphasizes simplicity and readability, making it accessible to beginners.
These features make Python versatile and widely used in web development, data analysis, artificial intelligence, scientific computing, and more.”
Q2. Explain the differences between lists and tuples in Python.
“In Python, both lists and tuples are used to store collections of items. The key differences between them are mutability, performance, and, of course, the syntax.
Lists are mutable, meaning we can modify their contents (add, remove, or change items). In contrast, Tuples are immutable; their contents cannot be changed once created. Therefore, Tuples is slightly faster than lists due to its immutability.
Lists are defined with square brackets [], while tuples are defined with parentheses ().
Q3. Can you tell me about the usage of Lists and Tuples in Python?
Lists are used when the data may change over time. Unlike lists, tuples are preferred for data that should remain constant throughout the program and can be used as keys in dictionaries.
Q4. How is memory managed in Python?
Python manages memory automatically through the use of a private heap containing all Python objects and data structures. The management of this private heap is ensured by the Python memory manager. The key components of Python’s memory management include automatic garbage collection, memory pools, and dynamic typing.
Python utilizes reference counting to detect and reclaim memory from unused objects, along with a cyclic garbage collector to free up circular references.
Moreover, Python organizes the memory in the heap into pools, which are blocks of memory for objects of similar size, managed by the “pymalloc” algorithm to minimize overhead.
Another important aspect is that in Python, the memory allocation and deallocation for objects are handled dynamically, ensuring efficient memory use and reducing memory waste.
Q5. What is the “pymalloc” algorithm?
The “pymalloc” algorithm is a specialized memory allocator used by Python to efficiently manage memory allocation and deallocation for small objects. It operates as part of Python’s memory management system to enhance performance, particularly for operations that frequently create and destroy many small objects, which is common in Python programs.
By using “pymalloc“, Python can manage memory for small objects more quickly and efficiently than relying on the general-purpose memory management provided by the operating system.
When Python needs to allocate memory for a small object (typically less than 256 bytes), “pymalloc” attempts to fulfill the request from a pool of memory blocks of the appropriate size.
If a suitable pool with free blocks is available, the memory is allocated from there. Otherwise, a new pool is allocated for that size class.
For objects that are too large to be managed by “pymalloc“, Python falls back on the system’s memory allocator, directly requesting memory from the operating system.
Q6. What are Python decorators, and how would you use them?
“Python decorators are tools for modifying the behavior of functions or classes without altering their code. They are implemented as wrappers around functions or methods, allowing us to add functionality before or after the target function runs, effectively enhancing or changing its behavior.
A decorator is applied to a function or method by prefixing its definition with the decorator’s name preceded by an @ symbol. For example, “@decorator_name“.
We can use them to log information about function arguments and execution time. We can also use them to check if a user has the necessary permissions to execute a function and to cache the results of expensive function calls.
Q7. Explain the difference between “deepcopy” and “shallowcopy” in Python.
In Python, “shallowcopy” and “deepcopy” are used to copy objects, but they do so in different ways:
Shallow Copy creates a new object but does not create copies of the objects found within the original object. Instead, it copies references to the same objects. This means changes to mutable objects within the copied object will reflect in the original object. A shallow copy is created using the “copy()” method or the “copy” module’s “copy()” function.
On the other hand, Deep Copy creates a new object and recursively copies all objects found within the original object. This means all objects within the copied object are also copies, not references to the original items. Changes to the copied object do not affect the original object. A deep copy is created using the “copy” module’s “deepcopy()” function.
In summary, use a shallow copy when we want to copy an object without duplicating the objects it contains and a deep copy when we need a completely independent copy of an object and all objects it contains.
Q8. What is PEP 8, and why is it important?
PEP 8, or Python Enhancement Proposal 8, is the official style guide for the Python programming language. It provides conventions for writing readable and consistent Python code. These conventions cover code layout, naming conventions, and programming recommendations to enhance code quality and team productivity.
PEP 8 ensures consistency by adhering to a common set of guidelines, developers can write code that others can more easily understand and maintain. It also improves readability by ensuring clear and consistent coding styles, so other team members can easily understand the code.
Q9. How would you manage packages in Python?
In Python, package management is primarily handled using “pip,” the package installer for Python, which allows us to install, update, and remove packages from the Python Package Index (PyPI) and other package indexes.
For more complex dependency management and project environments, we can use virtual environments and, when necessary, tools like “conda” for managing both Python and non-Python packages or “poetry” for dependency management and packaging.
Q10. Explain the use of the global and nonlocal keywords in Python.
In Python, “global” and “nonlocal” keywords are used to modify the scope of variables outside the current scope.
The “global” keyword is used to declare that a variable inside a function refers to a global variable. This allows functions to modify variables that are defined in the global scope.
Without “global“, the variable would be considered local to the function, and any assignment would define a new local variable.
On the other hand, the “nonlocal” keyword is used in nested functions to declare that a variable refers to a variable in the nearest enclosing scope that is not global. This allows the nested function to modify the variable.
Q11. What are Python generators?
Python generators are a type of iterable, like lists or tuples, but they lazily generate values on the fly without storing them in memory. This makes generators highly efficient for working with large datasets or infinite sequences, as they produce items one at a time and only as needed.
Generators are created using functions and the yield keyword. Instead of returning a value and exiting, a generator function automatically suspends and resumes its execution and state around the last point of value generation. The advantage of generators over lists is their ability to generate a sequence of values over time rather than computing the sequence all at once and holding it in memory
Q12. How do you use Python generators?
To use Python generators, first, we define a generator function by using the “def” keyword and “yield” instead of “return” to provide a value to the caller and pause execution
We can iterate over a generator by using a “for-loop” or the “next()” function for each iteration to produce the next value in the sequence.
Q13. How can you achieve concurrency in Python?
In Python, concurrency can be achieved through multiple mechanisms, each suited to different scenarios.
For example, for I/O-bound tasks where the program waits for external events, we can use threading, which utilizes threads to execute multiple threads concurrently in a single process.
For such tasks, we can also use Asynchronous Programming (asyncio), which allows a single thread to handle multiple tasks by waiting for I/O operations to complete without blocking.
For CPU-bound tasks that require parallel computation, we can use “Multiprocessing” to leverage multiple processes instead of threads, each with its own Python interpreter and memory space, thus bypassing the Global Interpreter Lock (GIL).
We can also use “Concurrent Futures,” which is a high-level interface for asynchronously executing callables using threads or processes to avoid the direct management of threads and processes for simple execution of functions concurrently.
Q14. Describe how you handle exceptions in Python.
In Python, we do exception handling by the use of “try-except” blocks to allow a program to handle errors and continue its execution or provide informative feedback to the user.
The “try” Block encapsulates the code that might raise an exception. So. if we suspect a code to cause an error, we put it in the “try” block.
We follow the “try” block with one or more “except” blocks to catch and handle specific exceptions. We can specify the type of exception to catch (e.g., “ValueError“, “TypeError“), or use a generic “except” to catch any exceptions.
As an option, after the “except” blocks, we can include an “else” block that runs if the code in the “try” block did not raise an exception.
Another option is to use a “finally” block to define cleanup actions that must be executed under all circumstances, such as closing files or releasing resources, regardless of whether an exception was raised.
Q15. What is list comprehension in Python?
List comprehension in Python is an efficient way to create lists. It allows us to generate new lists by applying an expression to each item in a sequence or iterable. It optionally filters items to include only those that meet a certain condition. It’s a more syntactically compact and readable alternative to using loops for list creation.
Q16. What are lambda functions in Python?
Lambda functions in Python, also known as anonymous functions, are small, one-line functions defined without a name using the “lambda” keyword.
A “lambda” function can have any number of arguments but only one expression, the result of which is returned by the function. Lambda functions are useful for simple operations that are easily defined in a single expression.
These are often used in situations where a function is needed temporarily for a short period, like as an argument to higher-order functions like “map()“, “filter()“, and “sorted()“.
Q16. How does Python’s garbage collection work?
Python’s garbage collection is primarily managed by the use of reference counting along with a cyclic garbage collector for detecting and collecting cycles.
Each object in Python has a reference count that tracks how many references point to the object.
When an object’s reference count drops to zero, meaning no references to it exist anymore, Python automatically deallocates or ‘garbage collects’ that object to free up memory.
However, reference counting alone can’t handle reference cycles (where two or more objects reference each other), as these objects’ reference counts may never reach zero. To solve this, Python uses a cyclic garbage collector that periodically searches for groups of objects that are only accessible by each other, thus enabling Python to reclaim memory from cycles.
Q17. Explain the GIL in Python and its implications.
The GIL, or Global Interpreter Lock, is a mechanism used in CPython (the standard Python implementation) to ensure that only one thread executes Python bytecode at a time.
This lock is necessary because CPython’s memory management is not thread-safe. The GIL prevents multiple native threads from executing Python bytecodes concurrently, which could lead to race conditions and corrupt data structures.
The main implication of the GIL is that it limits the execution of multi-threaded Python programs on multi-core processors. Even if a system has multiple cores, a Python program using threading is unable to run threads in parallel due to the GIL. Because of this, the threads are executed sequentially. This makes multi-threading in Python suitable for I/O-bound applications but less effective for CPU-bound tasks.
To overcome the limitations imposed by the GIL for CPU-bound tasks, Python developers can use multi-processing instead of multi-threading, which involves running code across multiple Python processes that are not affected by the GIL, thus enabling parallel execution on multiple cores.
Q18. Describe how you would implement a Python application to be thread-safe.
To implement a thread-safe Python application, I would follow several key practices to ensure that shared data is accessed and modified safely across threads. These practices include:
- Using Locks and Semaphores to utilize threading module constructs like Locks, RLocks, Semaphores, or Conditions to synchronize access to shared resources. This means acquiring a lock before accessing or modifying the resource and releasing the lock afterward.
- Avoiding Global states to minimize or manage access to global states within threads. When the global state is necessary, ensure access is controlled through synchronization mechanisms.
- By opting for thread-safe data structures provided by Python, such as queues from the “queue” module, which is designed to communicate safely between threads.
- Using immutable data structures, when possible, as they can’t be altered after creation, naturally avoiding many concurrency issues.
- Implement well-known concurrency patterns, such as the Producer-Consumer pattern, which can help structure the application in a way that reduces the complexity of thread interaction and data sharing.
- By using the multiprocessing module for CPU-bound tasks instead of threading. This module bypasses the Global Interpreter Lock (GIL) by using processes instead of threads, each with its own Python interpreter and memory space, thus avoiding the need for GIL.
Q19. What is a metaclass in Python, and why would you use it?
A metaclass in Python is a class of a class that defines how a class behaves. A class is an instance of a metaclass, just as an object is an instance of a class. Metaclasses are used to create classes with specific traits or behaviors, allowing for more dynamic and flexible object-oriented programming.
Metaclasses are a powerful, though complex, feature of Python and are generally used in framework or library development where such dynamic behavior is needed.
Metaclasses are used for advanced scenarios like:
- Intercepting Class Creation to modify or augment a class at the time of its creation.
- Controlling Class Instantiation to customize how classes are instantiated.
- Implementing Singleton Patterns to ensure a class has only one instance.
- Automatic Resource Management or Registration for automatically registering classes in a program or ensuring resources are managed consistently.
An example of using a metaclass might be automatically registering all subclasses in a registry to manage them centrally or enforcing certain attributes or methods in subclasses.
Q20. How can you manage state in a Python application?
Managing the state in a Python application can be achieved through various strategies, depending on the application’s complexity and requirements. Key approaches include:
- Global Variables: Global variables can hold “state” for simple scenarios. However, this approach should be used sparingly due to potential issues with code maintainability and testing.
- Classes and Objects: Using classes to encapsulate state and behavior. Objects of these classes can hold state across the application lifecycle, making it easier to manage and update as needed.
- Databases: For a persistent state that needs to survive between application restarts, databases (relational or NoSQL) can be used to store and retrieve the application state.
- Caching: In-memory data stores like Redis or Memcached can be used for fast, temporary state management, which is especially useful for web applications needing to manage session state.
- File System: Storing state in files (e.g., JSON, XML, or plain text) for persistence, useful for configuration or data that changes infrequently.
- Context Managers: Utilize Python’s context managers to manage resources and state in a structured manner, especially for managing resource acquisition and release patterns.
Each method has its use cases; often, a combination is used in a single application. The choice depends on the state’s nature, whether it’s temporary or persistent, complexity, and performance considerations.
Q21. Explain Python’s use of the “with” statement and context managers.
When used with context managers, the “with” statement in Python automatically manages the setup and teardown of resources. The “_enter_” method is called at the beginning of the block, initializing the context, and the “_exit_” method is invoked at the end, cleaning up the resources, regardless of whether the block was exited normally or due to an exception
For example, when working with files, the “with” statement ensures that the file is properly closed after its block is executed, even if exceptions are raised within the block
Q22. What is a Context Manager?
A context manager is an object that defines the runtime context to be established when executing a “with” statement. It handles the entry into and the exit from the desired runtime context.
This typically involves allocating and releasing resources. Context managers implement the “_enter_” and “_exit_” methods to define what happens when entering and leaving the context.
Q23. How do you debug a Python application? Describe the tools and techniques you would use.
To debug a Python application, I employ a combination of techniques and tools tailored to the nature of the issue. Key strategies include:
- Print Statements: Inserting “print()” statements to output the values of variables at specific points in the code. Although basic, it’s quick and effective for simple issues.
- Logging: Using Python’s built-in “logging” module to write debug information, warnings, and errors to a log file, which is more structured and flexible than print statements.
- Interactive Debuggers: Utilizing interactive debugging tools like “pdb” (Python Debugger) or “ipdb” (an IPython-enhanced version of “pdb“) to pause execution, inspect variables, and step through the code at runtime. This is particularly useful for complex issues.
- IDE Debuggers: Leveraging Integrated Development Environment (IDE) features, such as breakpoints, watch windows, and step-through execution. Tools like PyCharm or Visual Studio Code offer powerful debugging capabilities in a user-friendly interface.
- Static Analysis Tools: Using tools like PyLint or MyPy to detect syntax errors, type issues, and potential bugs before runtime.
- Unit Testing: Writing unit tests with frameworks like “unittest” or “pytest” to identify and isolate bugs in individual components of the application.
- Profiling Tools: For performance issues, profiling tools like “cProfile” can help identify bottlenecks in the code.
I combine these approaches based on the debugging context, starting with the simplest applicable method and escalating to more sophisticated tools as needed.
Q24. What are Python’s iterators, and how are they different from generators?
In Python, iterators and generators both allow us to iterate over data, but they do so in different ways.
Iterators are objects that implement the iterator protocol, consisting of the “_iter()_” and “_next()_” methods. “_iter()_” returns the iterator object itself and “_next()_” returns the next value from the iterator. When there are no more items, “_next()_” raises a “StopIteration” exception. Iterators can be created from iterable objects like lists or strings using the “_iter()_” function.
Generators are a simpler way to create iterators using a function with the “yield” keyword.
When the generator function is called, it returns a generator iterator. This iterator generates values one at a time from the function body by suspending and resuming execution each time “yield” is encountered.
Unlike a function that returns a list, a generator does not create all items at once; it generates them on the fly, which can be more memory efficient.
The key difference is that generators are a type of iterator specifically designed to be created through functions with “yield” expressions, providing a convenient and concise way to create lazy iterators. In contrast, any object that manually implements the “_iter()_” And “_next()_” methods can be an iterator, not necessarily related to generator functions.
Q25. How would you optimize the performance of a Python application?
To optimize the performance of a Python application, I’d take a systematic approach, focusing on identifying bottlenecks and choosing the right strategies to address them. Key steps include:
- Profiling: Use profiling tools like “cProfile” to identify where the application spends most of its time. This helps pinpoint performance bottlenecks.
- Algorithm Optimization: Review and optimize algorithms. Often, performance issues can be significantly mitigated by choosing more efficient algorithms or data structures.
- Avoiding Global Interpreter Lock (GIL) for CPU-bound tasks: Use multiprocessing instead of threading for CPU-bound tasks to take advantage of multiple CPU cores, as threading is limited by the GIL.
- Using Built-in Data Types and Functions: Python’s built-in types and functions are highly optimized. Where possible, use these instead of custom implementations.
- Utilizing Libraries: Leverage efficient third-party libraries like NumPy for numerical computations or Pandas for data analysis, which are optimized for performance.
- Minimizing Memory Footprint: For memory-intensive applications, manage memory usage by using generators instead of lists where appropriate and explicitly delete objects that are no longer needed.
- Cython or PyPy: Consider using “Cython” to compile Python to C for critical code paths or switch to an alternative Python interpreter like “PyPy” that can offer significant speed improvements for certain applications.
- Concurrency and Parallelism: Evaluate the application for opportunities to use concurrency (“asyncio” for I/O bound tasks) or parallelism (multiprocessing for CPU-bound tasks) to improve performance.
Performance optimization is context-dependent; the right approach depends on the specific performance issues and application requirements.
Q26. Describe a situation where you used Python’s multiprocessing module and the outcome.
In a previous project, we needed to process large datasets for data analysis, which was CPU-intensive and time-consuming when done sequentially. To optimize performance, I used Python’s “multiprocessing” module to parallelize the data processing.
I divided the dataset into chunks and used the “Pool” class to create a pool of worker processes, distributing chunks of data to these processes. This approach allowed the data processing to be executed in parallel across multiple CPU cores.
The outcome was a significant reduction in processing time. Tasks that initially took several hours to complete were reduced to a fraction of that time, greatly improving the efficiency of our data analysis pipeline and enabling us to handle larger datasets more effectively.
This experience demonstrated the power of parallel processing in Python and how the “multiprocessing” module can be leveraged to overcome the limitations of single-threaded execution, especially for CPU-bound tasks
Other Tech Interview Questions Lists
- Java Interview Questions
- JavaScript Interview Questions
- iOS Interview Questions
- Android Interview Questions
- NoSQL DB Interview Questions for Freshers
- NoSQL Interview Questions for Experienced
Articles about Python Programming
You may also like to read the following articles about Python:
- Python, from web development to machine learning
- Getting Started with Python
- Object-oriented Programming in Python
- NumPy for Data Science in Python
- pandas: An Ultimate Python Library for Data Science
- Python for Simplifying Data Operations in Data Science
- Data Visualization Using Python
- Data Engineering Interview Questions
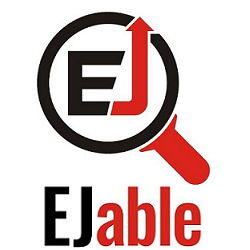
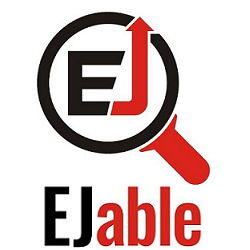