Getting Started with Python
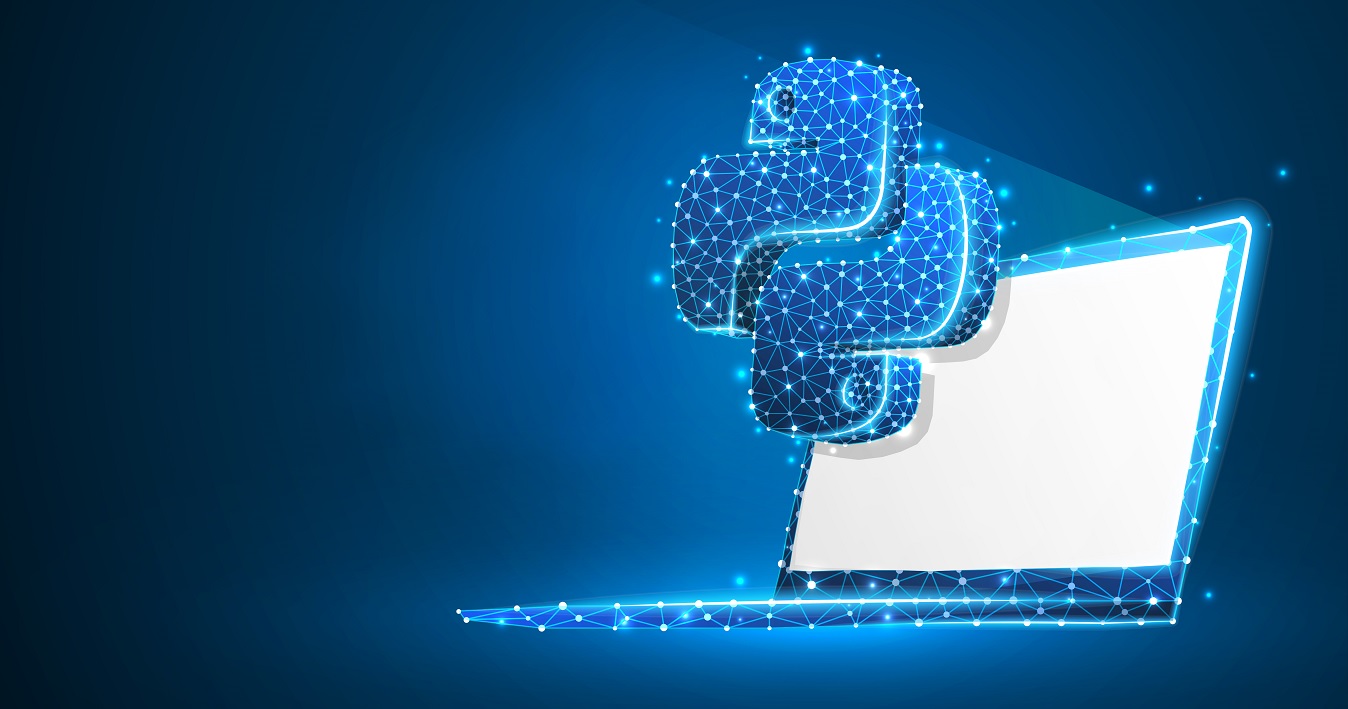
Python is a powerful programming language that provides many packages that we can use. Using the versatile Python programming language, we can develop the following:
- Automation
- Desktop application
- Android
- Web
- IoT home automation
- Data Science and the list goes on.
In this article, our primary focus will be knowing how to start learning Python and the essentials required to be a data scientist. Below is the list of topics covered in this post:
- Downloading and Installing Python on the system
- Executing Python Scripts
- Variables and types (Integer, Float, String, Boolean, None, Lists, Dictionaries)
- Type Hinting
- Escape Sequence
- List Slicing
- Looping over the list
- If/else blocks
- Truthy Values
- Exception Handling
- Other Data Types (‘Complex’, ‘bytes’ and ‘bytearray’, ‘tuple’, ‘set’ and ‘rozenset’)
Let’s get started!
Download Python
The first question is, “Which Python version should we use, Python-2 or Python-3?”
The newer version is always better, so it’s better to go for python-3. Though python-2 is maintained; however, no new features are added to it now. Whereas in Python-3, a lot of improvements are taking place.
Link from where you can download Python: https://www.python.org/downloads/
Python scripts
All the Python-related files are stored with the extension “.py”. This file contains a list of commands the Python compiler executes to achieve the desired results.
Let us create a simple index.py file. Below is the code where you can see that we have used #. It is used for adding comments to the Python file. “print” is the function to output our results. Let’s look at the following Python code:
#index.py
print(2 + 3)
print(4/ 10)
print(2* *4) # ** is for exponential
#output: 5
0.4
16
As you can see above, we have performed basic arithmetic operations. The shell where the Python code is executed is called “IPython” Shell.
NOTE: Using the Jupyter Notebook while solving Machine Learning/Data Science related problems is recommended. Please find the steps to set up the Jupyter Notebook.
Variable and Types
With Python, it is not necessary to create types.
In Python, we can save our data in a variable, as shown below:
score = 32.7
And if we want to check the type of our variable, we can use the following:
score = 32.7
type(score)
# output
float
Below are the data types that Python supports:
- Integer
- Float
- String
- Boolean
- None
- Lists
- Dictionaries
Type Hinting
As we know, there is no need to define types in Python while declaring a variable. But sometimes, from a development perspective, it is helpful to add type hinting to avoid runtime errors. Look at the example shown below:
def add_number ( x : int, y : int) ->int :
return a+b
Here in the above code, if we try to call the function as “add_number(5, “name”),” our IDE will start complaining even before the code is executed.
So, providing hints to our codebase is always preferred to avoid run-time errors.
We are now all good and set to explore different data types that Python supports.
Integer and float in Python
As we have seen above, we can define integer and float in Python simply as:
id=3
pi = 3.14
To convert an integer to float or from float to integer, we can do it as shown below:
int(3.14)#3
float(3) # 3.0
Strings
We can declare a string in Python as shown below:
name = "John"
There are a lot of methods that exist in the string:
"hello".capitalize() # Hello - Capitalize first letter of string
"hello".replace('e','a') # hallo
"hello".isalpha() # True
"123".isdigit() # True
"hello".upper() #HELLO "Hello".lower() # hello
# We can also use lstrip() or strip() function to remove left and right spaces respectively " Hello ".strip() # hello
'hello,hi, there'.split(',') # ['hello','hi','there']
String format
"Hello {0}".format("Python") # Hello Python
We can even format our string to display it in a way we want, as shown below:
Another format:
name = 'Python'
f"Hello {name}"
# output
Hello Python
Escape Sequence in Python
Like any other programming language, Python also has an escape Sequence. E.g., new line. Refer to the code shown below:
description = 'I am learning\ nPython' # \ n for new line
print(description)
#output
I am learning
Python
Follow the link to learn the whole list of escape sequences that Python supports.
What if we don’t want to treat backslash in the string as anything special? In such a scenario, we prefix our string with r. Consider an example shown below:
description = r'I am learning\ nPython'
print(description)
#output
I am learning\ nPython
Boolean
Boolean is simply binary with either True or false values. And in Python, we can define a variable as Boolean:
valid = True
invalid = False
None
We also have a data type in Python known as None. If we have some variable whose value is unknown, we can define it as None.
name = None
Lists
In Python, we can add multiple types to the list. Refer to the code shown below:
name = ['name1', 'name2']
print(name[0]) # name1
name.append('name3') # appended at end of list
name.remove('name3') # remove element from the list
'name1' in name # True
len(name) # gives length of the list
In the example shown above, note that we use the “append()” and “remove()” functions to add and remove the element from the list. We can also treat the list as a stack (LIFO)” and queue (FIFO). Refer to the example of the code shown below:
name = ['name1', 'name2', 'name3', 'name4']
name.pop() # It will remove element from the last. i.e. Stack
name.pop(0) # It will remove element from the beginning of the list. i.e.
Queue print(name)
# Output ['name2', 'name3']
We can even delete the value from the list, as shown below:
del name[0]
List Slicing
If we have a requirement where we need to do functions only with the part of the string, then we can do it as shown below:
name[1:] # skips first element
name[1:-1] #skips first and last element in the list
NOTE: When we are slicing the list in a way, name[1,2] name[1] is included in the list, whereas name[2] will not be included in the list.
Looping
To loop over all elements of the list, we can use for loop.
names =['john', 'rohit', 'jass']
for name in names:
print(name)
A range is a function that defines how many times we want our loop to execute. In the example shown below, the loop will be executed five times.
for index in range(5):
print(index)
Range function can also accept three parameters:
- Start index
- End index
- Increment
For example:
Range (5,10,2) will generate a list as [5,7,9] to iterate.
If we need a counter to iterate over the list, value, and index, then we can use ‘enumerate()‘ function.
names =['john', 'rohit', 'jass']
for i,a in enumerate(names) :
print('student ' + str(i) + ' name is '+ str(a))
# output:
student 0 name is john
student 1 name is rohit
student 2 name is jass
Dictionaries
Dictionaries allow us to store key-value pairs similar to a JSON object.
student = {
"name": "Python",
"code" : 123,
"valid" : True
}
If we want all the keys of the dictionary or a list of keys, we can get by:
print(student.keys())
print(list(student.keys()))
And if we want to check whether the key exists in the dictionary, we can use the following:
print('name' in student)
#output
True
If we want all the data in the dictionary, we can get it by the following:
student.values()
We have two different ways, as shown below, to get value based on the key from the dictionary:
student = { "name": "Python", "code" : 123, "valid" : True }
print(student['name'])
print(student.get('name'))
print(student.get('name', 'NA'))
The advantage of using the “get()” method is that the code won’t break and return None if the key doesn’t exist.
We use the “items()” function to iterate over the dictionary’s values. Refer to the code shown below:
student = { "name": "Python", "code" : 123, "valid" : True }
for key, val in student.items():
print(key, val)
# Output
name Python
code 123
valid True
To delete both key and value from the dictionary:
del student['name']
List dictionaries can also have multiple data types.
We can also create a list of dictionaries.
students = [
{"name": "Python", "code" : 123, "valid" : True},
{"name": "Post", "code" : 12, "valid" : False}
]
If/else Logic
Python uses indentation instead of curly braces for defining code blocks.
Example showing the ‘if/else’ logic:
name = 'Python'
if name == 'Python':
print('I am python')
elif name == 'Learing Python':
print('I am Learing Python')
else:
print('I am not python')
Instead of nesting ‘else‘ and ‘if‘, Python provides a combined version, ‘elif‘.
Truthy values
We can also perform Boolean check operations in the if loop, and based on the truthy results and the expressions are evaluated, Let’s look at the example shown below:
number = 10
if number and number > 5:
print('I am defined')
Exception handling
We have explored different data types that are there in Python. It’s always a good practice to handle errors in code so that our code doesn’t break if something goes wrong. So, let’s explore how to handle the errors.
Suppose we have a student dictionary as defined below:
student = {
"name": "Python",
"code" : 123,
"valid" : True
}
And we try to access a key that doesn’t exist, as shown below:
student['firstName']
It will result in an error. But wait, we can handle these errors as shown below:
try:
student['firstName']
except KeyError as error:
print("error occurred while accessing firstName")
print(error)
In the above case, we are specifically catching only “KeyError,” but what if we don’t know what kind of error will occur in the application? Then how will we handle that?
We can use “Exception” instead of “KeyError” to handle all the errors. Also, we can improve our above code in a way as shown below:
try:
student['firstName']
except Exception:
print("error occurred while accessing firstName")
We do have some other data types in Python 3 as well like:
- Complex
- bytes and ‘bytearray’
- tuple
- set and frozenset
Let’s look at them in detail:
‘Complex’ Data Type in Python
The complex is the data type to store complex numbers. Syntax to define complex numbers is as shown below:
complex(3,5) # where 3 is the real part, and 5 is the imaginary part of a complex number
The default value of the imaginary part of the complex number is zero.
‘bytes’ and ‘bytearray’ Data Types
“Bytes” and “bytearray” are almost similar. The only difference between “bytes” and “bytearray” is that “bytearray” is mutable, and “bytes” is immutable.
Let’s look at how to create a byte:
arr = [1,2,30] # Array which we need to convert to byte
byt = bytes(arr) # Converted to byte
list(byt) # To convert bytes to original data
Code to declare ‘bytearray’ Data Type:
arr = [1,2,30] # Array which we need to convert to bytearray
byt = bytearray(arr) # Converted to bytearray
list(byt) # To convert bytearray to original data
‘Tuple’ Data Type
We also have a data type called ‘tuple’. A tuple is like a ‘list’ but is immutable (It cannot be changed once created). Refer to the code shown below to declare the ‘tuple’ data type:
tup = (1,4, "Python")
And we can access a value from the “tuple” as shown below:
tup = (1,4, "Python")
print(tup[0]) #1
print(tup[2]) # Python
We can also convert ‘tuple’ to the data type ‘list’. Refer to the code shown below:
print(list(tup))
‘set’ and ‘frozenset’ Data Types
“Set and the “frozeset” are also like “list,” but they have only unique values. The difference between “set” and “frozenset” is that; “set” is mutable, whereas “frozenset” is immutable.
Let’s look at the sample code to declare ‘set’ as shown below:
set([1,1,'name','Python',4, 'Python'])
# output
{1, 'name', 4, 'Python'}
We can also perform all the “set” related operations like “union (|)”, “intersection (&)”, “difference (-)”, “symmetric difference (^)” etc. Refer to the code shown below:
A = {0, 2, 4, 6, 8}
B={1, 2, 3, 4, 5}
print("Union :", A | B) # Or, A.union(B)
print("Intersection :", A & B) # Or, A.intersection(B)
print("Difference :", A - B) # Or, A.difference(B)
print("Symmetric difference :", A ^ B) # Or, A.symmetric_difference(B)
NOTE: The symmetric difference between sets A and B is the set of elements in either sets A or B but not in both.
Let’s look at the sample code to declare ‘frozenset’ as shown below:
frozenset([1,1,'name','Python',4, 'Python'])
# output
{1, 'name', 4, 'Python'}
I hope this post is helpful to start learning Python programming. Happy Learning!!
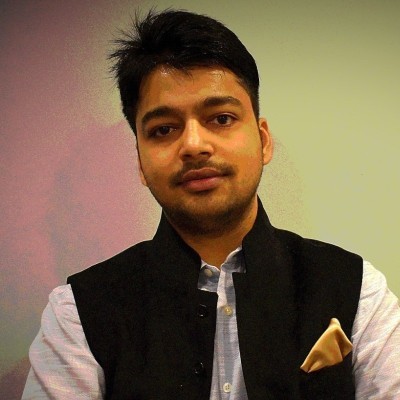
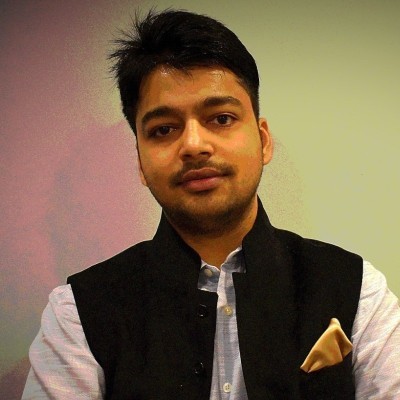
Tavish lives in Hyderabad, India, and works as a result-oriented data scientist specializing in improving the major key performance business indicators.
He understands how data can be used for business excellence and is a focused learner who enjoys sharing knowledge.