Android: Interview Questions and Answers
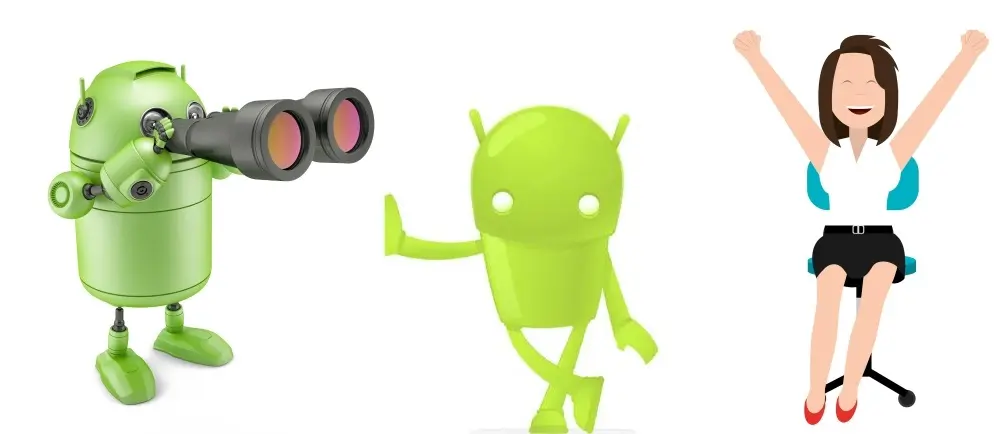
Here is a list of common Android interview questions with detailed answers to help you prepare for the interview as an Android app developer.
This article is designed to assist aspiring Android developers with a comprehensive set of interview questions, covering a wide spectrum of areas including basic programming principles, Android-specific components, design patterns, and best practices in mobile development.
Android Developer Interview Questions and Answers
Q1. Explain the Android application architecture and its main components.
Android application architecture is designed to simplify the development of complex applications by dividing the application into distinct layers, each with its specific role. At its core, the architecture consists of four main components: Activities, Services, Broadcast Receivers, and Content Providers.
- Activities: These are the entry points for interacting with the user. Each activity represents a single screen with a user interface. For example, an email application might have one activity to show a list of new emails, another activity to compose an email, and another to read emails.
- Services: These run in the background to perform long-running operations or to work on remote processes without a user interface. For instance, a service might play music in the background while the user is in a different application.
- Broadcast Receivers: These are components that enable the application to listen for and respond to broadcast messages from other applications or from the system itself. For example, an application can initiate a notification when the device starts by listening to the BOOT_COMPLETED broadcast.
- Content Providers: These manage a shared set of application data. Through content providers, other applications can query or even modify data (if the content provider allows it). For instance, the Contacts application uses a content provider to share contact data across different applications.
The Android App’s Manifest file (“AndroidManifest.xml”) declares the components and their capabilities, like permissions. Additionally, Android apps can include components like Fragments, “ViewModels”, and “LiveData” to manage UI components, data, and app logic more efficiently, following the recommended app architecture guidelines from Google.
Q2. What is the significance of the manifest file in Android?
The AndroidManifest.xml file serves as a central configuration file for Android applications. It performs several critical functions:
- Declares Application Components: It lists all components of the application, including activities, services, broadcast receivers, and content providers. This declaration is necessary for the Android system to recognize these components.
- Defines Permissions: The manifest specifies the permissions the application requires to access protected parts of the API and interact with other apps or the system. It also declares any permissions it offers to other apps.
- Application Requirements: It details the hardware and software features the application requires, such as a camera or internet access, which Google Play Store uses to filter out devices incompatible with the app.
- Intents: Through intent filters declared in the manifest, components can handle specific types of intents, enabling them to respond to actions from other apps or the system.
- App Metadata: It includes additional metadata about the application, such as the minimum Android version supported, application icon, theme, and more.
Therefore, the AndroidManifest.xml file is essential for defining the structure and metadata of an Android application, ensuring the system can properly manage and execute its components.
Q3. How does the Android operating system handle memory management?
Android’s memory management is designed to ensure that applications run smoothly on devices with limited memory. The system employs several strategies to manage memory, including:
- Automatic Garbage Collection: Android uses garbage collection to automatically reclaim memory used by objects that are no longer needed, reducing the risk of memory leaks.
- Memory Trim Level Notifications: The system notifies running applications of the current memory state through trim level notifications, encouraging them to release unused resources.
- Application Lifecycle Management: Android controls the lifecycle of applications, pausing or stopping activities that are not in the foreground to free up resources for the active application.
- Dalvik/ART Runtime: Earlier versions of Android use the “Dalvik” runtime, which is optimized for limited memory. Newer versions use the Android Runtime (ART), which further improves memory management and application performance through “ahead-of-time” (AOT) compilation.
Through these mechanisms, Android ensures that devices manage memory efficiently, prioritizing foreground applications while gracefully handling low-memory situations to maintain system stability.
Q4. What is an “Intent” in Android?
An “Intent” in Android is a messaging object used to request an action from another app component. It serves as a versatile communication tool within the app and between different apps. There are two main types of Intents:
- Explicit Intents specify the exact component to start by providing the fully qualified class name. They’re commonly used for internal app communications, such as starting a new activity within the same app.
- Implicit Intents do not directly specify the Android component to start. Instead, they declare a general action to perform, allowing any app component that can handle the action. This is typically used for system actions, like opening a URL in a web browser or sharing content between apps.
Intents can also carry data using key-value pairs, known as extras, to provide additional information required for the action to be performed.
Q5. Are there any other types of intents also?
In Android development, the primary categorization of Intents falls into two types: Explicit and Implicit Intents, as mentioned earlier.
However, beyond this basic classification, Intents can be further be described based on how they are used or their specific purposes, rather than being distinct types by themselves. Here’s a brief overview of these uses or characteristics that sometimes lead to informal categorizations:
Sticky Intents: These are a special kind of broadcast Intents that stick around after the broadcast is finished. An example is the battery status Intent (“ACTION_BATTERY_CHANGED“). When you register a receiver for a sticky Intent, you immediately receive the Intent with the last broadcast state, even if you haven’t registered the receiver at the time of the broadcast. Note that as of API level 21 (Android 5.0 Lollipop), registering for sticky broadcasts has been deprecated for implicit broadcasts due to performance considerations.
Pending Intents: While not a different type of Intent, a PendingIntent is a token that you give to a foreign application (e.g., “NotificationManager“, “AlarmManager“, Home Screen “AppWidgetManager“, or other third-party apps), which allows the foreign application to use your application’s permissions to execute a predefined piece of code. If you have an Intent to launch an activity, you can wrap it in a “PendingIntent” for use with a notification or widget.
Broadcast Intents: This refers more to the usage of Intents to broadcast events to multiple app components simultaneously, especially using the “sendBroadcast()“, “sendOrderedBroadcast()“, and “sendStickyBroadcast()” methods. Although this is not a separate type of Intent, it is a significant use case where Intents facilitate communication between different parts of your application or with other applications on the device.
In practical terms, the classification into Explicit and Implicit Intents covers the main types of Intents in Android. Other terms like Sticky Intents or Pending Intents describe specific use cases or ways to use Intent objects within the Android framework rather than being distinct types by their nature.
Q6. Explain the lifecycle of an Android Activity.
The lifecycle of an Android Activity is a set of states through which an Activity passes from the time it is created until it is destroyed. These states are managed by a series of callback methods within the Activity class:
- “onCreate()“: The system calls this method when creating the Activity. Here, you perform initial setup, such as creating views and binding data to lists. This method receives the parameter Bundle savedInstanceState, which contains the activity’s previously saved state.
- “onStart()“: This method is called when the Activity becomes visible to the user. It follows “onCreate()” and precedes onResume().
- “onResume()“: Called when the Activity starts interacting with the user. At this point, the Activity is at the top of the activity stack and captures all user input. It follows “onStart()” or “onPause()“.
- “onPause()“: The system calls this method the first indication that the user is leaving the Activity (though it does not always mean the activity is being destroyed); it indicates that the activity is no longer in the foreground (though it may still be visible if the user is in multi-window mode). Use “onPause()” to pause or adjust operations that should not continue (or should continue in moderation) while the Activity is in the Paused state and that you expect to resume shortly.
- “onStop()“: Called when the Activity is no longer visible to the user, either because another activity has taken over or because the activity is being destroyed. It follows “onPause()” or may be followed by onRestart() if the activity comes back to the foreground or “onDestroy()” if the activity is going away.
- “onRestart()“: Called after the Activity has been stopped, just prior to it being started again. Always followed by “onStart()“.
- “onDestroy()“: The final call that the Activity receives. This can be either because the activity is finishing (someone called “finish()” on it), or the system is temporarily destroying this instance of the Activity to save space.
Q7. How do you persist data in an Android app?
In Android, there are several ways to persist data, depending on the type and amount of data, as well as the specific needs of the application:
- Shared Preferences: Ideal for storing small amounts of data in key-value pairs, such as user settings or preferences. Shared Preferences are simple to use and can store primitive data types.
- SQLite Databases: Suitable for structured data storage, SQLite databases provide a robust solution for storing larger amounts of data that can be queried. Android offers the Room Persistence Library as an abstraction layer over SQLite to streamline database access while leveraging SQLite’s full power.
- Internal & External Storage: For storing files and media, apps can use Android’s internal storage (private to the app) or external storage (shared space). This is useful for documents, photos, or large datasets that don’t fit well into a database or key-value store.
- Room Database: Part of the Android Jetpack suite, Room provides an abstraction layer over SQLite. It simplifies database work and allows for more complex data storage and retrieval operations with less boilerplate code.
- DataStore: A newer data storage solution that addresses some limitations of SharedPreferences by offering a more robust and flexible way to store data asynchronously, with support for typed objects and data validation.
Q8. What is a Service in Android, and how is it different from an Activity?
In Android, a Service is a component that performs long-running operations in the background without providing a user interface. It can continue to run even when the user switches to another application. Services are used for tasks such as playing music, handling network transactions, performing file I/O, or interacting with content providers, all running in the background.
Differences between a Service and an Activity include:
- User Interface: Activities are the primary components for creating a user interface. Users directly interact with Activities through the screen. Services, on the other hand, do not have a UI and run in the background to perform work for the application.
- Lifecycle: An Activity has a complex lifecycle controlled by user interactions (e.g., starting, pausing, resuming, and stopping). A Service has a simpler lifecycle; it is started and can run in the background indefinitely, even if the user is interacting with another app.
- Purpose: Activities are intended to facilitate user interaction, serving as the entry point for users to perform actions and view data. Services are designed for background operations that should continue without direct user interaction.
Therefore, while Activities interact with users through the UI, Services work in the background to perform operations even when the user is not directly interacting with the application.
Q9. What is a Fragment in Android development?
A “Fragment” in Android is a modular section of an activity, which has its own lifecycle, receives its own input events, and which you can add or remove while the activity is running.
Fragments are used to build dynamic and flexible user interfaces.
Unlike an activity, a fragment cannot exist independently; it must be hosted by an activity or another fragment.
The key advantages of using fragments include the reusability of components across different activities, adaptability to different screen sizes and orientations, and simplified management of user interfaces in large-scale applications.
Fragments support their own layout, lifecycle, and handling of user interaction events, allowing developers to organize their code better and more efficiently manage UI components.
Q10. How can you communicate between two Fragments?
To communicate between two Fragments in an Android application, using a shared “ViewModel” or the host Activity is recommended as an intermediary. Here’s a brief overview of each method:
Shared “ViewModel“: By using a ViewModel that is scoped to the activity hosting the fragments, both fragments can access and modify the same data. The “ViewModel” acts as a shared data holder. Since both fragments observe the data in the “ViewModel“, when one fragment updates the data, the other fragment can react to these changes automatically.
Via the Host Activity: Each fragment communicates through the host activity. One fragment sends data to the activity, which then passes the data to the other fragment. This involves defining an interface in the fragment and implementing it within the activity to facilitate communication.
Q11. Explain Android’s Intent Filter.
An “Intent Filter” in Android is a way of declaring the capabilities of a component, such as an Activity, Service, or Broadcast Receiver. It specifies the types of intents that a component is willing to accept. By declaring an intent filter, a component indicates to the Android system which implicit intents it can handle based on the action, data, and category specified in the intent.
For example, an Activity might declare an intent filter for viewing URLs, indicating it can handle intents to display web pages. When an app sends an intent that matches the filter, the Android system may start the component, if it’s not already running, and deliver the intent.
Intent filters are crucial for inter-component communication and app integration, allowing components from different apps to seamlessly work together without explicit class names, promoting a more flexible and modular application architecture.
Q12. What are Android Jetpack components?
Android Jetpack is a suite of libraries, tools, and guidance to help developers follow best practices, reduce boilerplate code, and write code that works consistently across Android versions and devices. Jetpack components are divided into four main categories:
- Foundation components provide basic functionality like backward compatibility, testing, and Kotlin language support. Examples include “AppCompa“t, “Android KTX“, and “Test“.
- Architecture components help you design robust, testable, and maintainable apps. They include “LiveData“, “ViewModel“, “Room“, and “Data Binding“.
- Behavior components assist in managing UI components and handling user interactions. Examples are Download Manager, Media & Playback, Notifications, and Permissions.
- UI components provide widgets and helpers to make your app not only easy to use but also beautiful. Examples include Animation & Transitions, Fragment, Layout, and “EmojiCompat“.
Q13. How do you implement background tasks in Android?
To implement background tasks in Android, we primarily use the WorkManager API for deferrable tasks requiring guaranteed execution. “WorkManager” is part of the Android Jetpack suite and is designed for tasks that can run even if the app exits or the device restarts. Here’s a brief overview:
- Define a WorkRequest: You encapsulate your task in a WorkRequest, which describes when and under what conditions your task should run. “WorkManager” supports one-time and periodic “WorkRequests“.
- Create a Worker class: Implement your task in a Worker class by overriding the “doWork()” method. This method runs asynchronously on a background thread provided by “WorkManager“.
- Enqueue the WorkRequest: With your “WorkRequest” configured, you enqueue it with WorkManager. WorkManager takes care of running your task according to its constraints and retry policy.
For real-time operations, developers might use services like Foreground Services for tasks that need immediate execution and should be noticeable to the user, ensuring the system does not kill the service.
Q14. What is the difference between a File, a Class, and an Activity in Android?
- File: A file is a unit of storage managed by the file system. It’s used to store data, such as text, images, or binary data, and can be read from or written to by your application. In Android, files can be stored internally (private to your app) or externally (accessible by other apps).
- Class: A class is a blueprint or template in Java or Kotlin from which objects are created. It defines properties (data) and methods (functions or behavior) that the objects created from the class will have. In Android, classes are used to define components such as activities, services, and more, as well as to create custom data types and logic.
- Activity: An Activity is a specific class in Android that provides a screen with which users can interact in order to do something, such as dial the phone, take a photo, send an email, or view a map. Each activity is given a window in which to draw its user interface. It’s one of the fundamental building blocks of an Android application’s user interface, and the lifecycle of an activity is managed by the Android OS.
Q15. How do you ensure your Android app is accessible for persons with disabilities?
Ensuring an Android app is accessible involves implementing design and development practices that accommodate users with disabilities. Key practices include:
- Use of Content Descriptions: For interactive elements and images, adding content descriptions using the android:contentDescription attribute helps screen readers announce the purpose of the elements, making the app usable for visually impaired users.
- Contrast and Text Size: Ensuring high contrast between text and its background and supporting dynamic text sizes allows users with visual impairments to read content more easily.
- Touchable Target Size: Making sure touch targets for buttons and other interactive elements are at least 48dp in height and width facilitates easier interaction for users with motor impairments.
- Keyboard Accessibility: Ensuring the app is navigable using a keyboard or directional controls benefits users who cannot interact with the app through touch alone.
- Testing with Accessibility Tools: Utilizing tools like TalkBack (screen reader) and Accessibility Scanner to test the app helps identify and rectify accessibility issues.
- Following the Accessibility Guidelines: Adhering to the Web Content Accessibility Guidelines (WCAG) and Android’s accessibility guidelines ensures a broad range of users can effectively use the app.
Q16. What are some common performance issues in Android apps, and how do you address them?
Common performance issues in Android apps include memory leaks, slow UI rendering, and excessive battery usage. To address these issues, one can adopt the following strategies:
- Memory Leaks: Use tools like “LeakCanary” to detect and fix leaks. Ensure that context-heavy objects are not kept longer than necessary and that static fields are used judiciously.
- Slow UI Rendering: Optimize layout hierarchies by reducing nesting, using “ConstraintLayout” for complex layouts, and ensuring smooth animations and transitions. The Android Profiler and Layout Inspector in Android Studio can help identify bottlenecks.
- Excessive Battery Usage: Monitor and optimize background tasks to minimize wake locks and unnecessary processing. Use JobScheduler for deferrable background tasks and ensure efficient use of network operations with batching and caching.
- Network Performance: Minimize data usage and optimize network calls by using caching strategies, compressing data, and prefetching data intelligently.
- Thread Management: Use background threads, “AsyncTask“, or Kotlin coroutines for long-running operations to keep the UI thread responsive.
Q17. Explain the concept of Material Design.
“Material Design” is a design language developed by Google, introduced in 2014. It aims to create a visual language that synthesizes classic principles of good design with the innovation and possibility of technology and science. Material Design is grounded in tactile reality, inspired by the study of paper and ink, yet technologically advanced and open to imagination and magic.
Key principles include:
- Realistic Visual Cues: It uses shadows, lighting, and motion to mimic the physical world and convey how elements are organized and interact with each other.
- Bold, Graphic, Intentional: Material Design emphasizes bold colors, typography, and deliberate white space to create hierarchy, meaning, and focus.
- Responsive Interaction: It provides feedback in response to user interactions, making the user feel directly engaged with the interface.
- Unified Experience Across Platforms: It seeks to create a consistent user experience across devices of different sizes and capabilities, from watches to desktops.
Material Design guidelines offer comprehensive advice on layout, color, typography, icons, and motion, helping developers and designers create visually appealing and functionally rich applications.
Q18. How do you manage app dependencies with Gradle?
Managing app dependencies with Gradle involves specifying them in your project’s build.gradle files. Gradle is Android’s build system that automates dependency management, among other tasks. Here’s a brief overview of the process:
- Declare repositories: First, specify the repositories in your “build.gradle” file where Gradle should look for the dependencies. Common repositories include Google’s Maven repository and JCenter.
- Add dependencies: Then, in your module-level “build.gradle” file, add dependencies by specifying the library’s group ID, artifact ID, and version number within the dependencies block.
- Sync the project: After adding or updating dependencies, sync your project with the Gradle files. This process downloads the specified libraries from the repositories and includes them in your project, making their APIs available for use in your app.
- Manage transitive dependencies: Gradle automatically resolves transitive dependencies (dependencies of your dependencies) but provides options to exclude specific ones if necessary.
- Use variables for versions: To keep dependencies organized, especially versions, you can define them in the project-level “build.gradle” file and reference them in module-level files.
By following these steps, developers can efficiently manage and update their app’s dependencies, ensuring that they incorporate the latest versions of libraries and tools essential for their application.
Q19. Explain the use of “ViewModel” in Android.
The “ViewModel” is a component of the Android Architecture Components designed to store and manage UI-related data in a lifecycle-aware manner. This means that the “ViewModel” holds the data for the UI and communicates with the model to fetch and store the data. It survives configuration changes such as screen rotations, ensuring that data is not lost during these events.
The primary purposes of using a “ViewModel” include:
- Separation of Concerns: By handling UI data operations within the “ViewModel“, it keeps the UI controllers (such as activities and fragments) clean and focused on rendering the UI and handling user interactions.
- Data Persistence: The “ViewModel” persists data across configuration changes, avoiding unnecessary network requests or database queries.
- Lifecycle Awareness: The “ViewModel” is aware of the lifecycle of its related UI controller. It automatically cleans up the resources when the UI controller is destroyed, preventing memory leaks.
To use a “ViewModel“, we instantiate it in our activity or fragment and observe “LiveData” within it. “LiveData“, also part of the Android Architecture Components, allows the UI to observe changes in the data stored in the “ViewModel“, enabling reactive UI updates.
Q20. How do you handle configuration changes in Android?
Handling configuration changes in Android, such as screen rotations, involves ensuring that the UI state and data are preserved. The primary strategy is to use the “ViewModel” component from the Android Architecture Components. ViewModel stores UI-related data that isn’t destroyed on configuration changes. Here’s how it works:
- Using ViewModel: We store our UI data in a ViewModel associated with the activity or fragment. When a configuration change occurs, such as a screen rotation, the ViewModel survives and is automatically re-associated with the new instance of the activity or fragment. This way, our UI data remains intact.
- “onSaveInstanceState()“: For small amounts of data and to manage UI state, override the “onSaveInstanceState(“) method in our activity or fragment. We Save our state to the Bundle provided in onSaveInstanceState(). Android system passes this Bundle back to your activity or fragment after the configuration change, which we can use in “onCreate()” or “onRestoreInstanceState()” to restore your state.
- Handling with Fragments: We can also manage configuration changes by retaining an instance of a fragment across configuration changes using “setRetainInstance(true)“. This approach keeps the fragment instance in memory, preserving its state.
These strategies allow us to maintain a seamless user experience by preserving data and state across configuration changes in Android applications.
Q21. What is Dependency Injection and how is it implemented in Android?
“Dependency Injection” (DI) is a design pattern that allows a class to receive its dependencies from an external source rather than creating them internally. This promotes decoupling, enhances testability, and makes the code more modular and maintainable.
In Android, DI can be implemented manually, but it’s more commonly managed through libraries like Dagger or Hilt, which automate and streamline the process.
- Dagger: A fully static, compile-time DI framework that generates injection code at compile time. It requires manual setup of components and modules to declare dependencies. Dagger provides flexibility and efficiency but with a steeper learning curve.
- Hilt: Built on top of Dagger, “Hilt” is a DI library for Android that simplifies Dagger-related infrastructure for Android apps. By leveraging predefined components and scopes, Hilt reduces boilerplate, making it easier to implement DI. Hilt automatically generates Dagger components, making it easier to manage dependencies across the application.
To use Hilt, for example, we annotate our application class with “@HiltAndroidApp“, activities, and fragments with “@AndroidEntryPoint“, and use “@Inject” to annotate constructor parameters, fields, or methods where dependencies should be injected.
Q22. Describe how to use Retrofit for network operations in Android.
Retrofit is a type-safe HTTP client for Android (and Java) developed by Square. It simplifies the process of making network requests by turning your HTTP API into a Java interface. Here’s a brief overview of how to use Retrofit:
- Define the HTTP API: Create a Java interface to define the HTTP methods (“GET“, “POST“, etc.) along with the endpoints. Use annotations to specify the request type and parameters.
- Create a Retrofit Instance: Instantiate a Retrofit object using the Retrofit.Builder(), specify the base URL for the web service and add a converter factory to serialize and deserialize objects, commonly Gson for JSON.
- Create a Service Instance: Use the Retrofit instance to create the service interface implementation.
- Make Asynchronous Requests: Call the methods defined in the interface to execute network operations asynchronously, handling responses and failures within callback methods.
Retrofit handles the HTTP connection and serialization, simplifying network operations and result parsing in Android applications.
Q23. Explain the difference between “runOnUiThread()” and “AsyncTask”.
“runOnUiThread()” and “AsyncTask” are both mechanisms in Android for managing background tasks and UI updates, but they serve different purposes and operate differently:
- “runOnUiThread()“: This method is used to execute a block of code on the main UI thread from a background thread. It’s specifically used for making UI updates or performing short operations that need to run on the UI thread. Since direct manipulation of UI elements from a background thread is not allowed, “runOnUiThread()” provides a way to safely interact with the UI after completing background work.
- “AsyncTask“: AsyncTask is a deprecated Android utility class (as of API 30) that simplifies running operations in the background and publishing results on the UI thread without having to manually handle threads or handlers. It defines three main types of generic parameters: Params, Progress, and Result, and provides callback methods like “doInBackground()“, “onProgressUpdate()“, and “onPostExecute()” to perform background tasks and post results to the UI thread.
While “runOnUiThread()” is a straightforward way to perform quick UI updates from a background thread, “AsyncTask” offers a more structured approach for handling background tasks with the ability to publish progress and results to the UI thread. However, due to its limitations and potential for memory leaks, developers are encouraged to use more modern approaches like “java.util.concurrent” classes, LiveData, and Kotlin Coroutines for background processing and UI updates.
Q24. How do you use LiveData in Android development?
The “Singleton” design pattern ensures that a class has only one instance and provides a global point of access to it. In Android development, Singletons are often used for scenarios where a single, globally accessible object is needed to manage shared resources or coordinate actions across the application, such as managing database connections or shared preferences.
Implementing a Singleton in Android involves:
- Private Constructor: Make the class constructor private to prevent instantiation from outside the class.
- Static Instance: Create a static instance of the class within itself.
- Public Static Method: Provide a public static method that returns the instance of the class, creating it if necessary.
However, it’s crucial to consider thread safety and memory leaks when implementing Singletons in Android. Using synchronized blocks or methods ensures thread safety, while being cautious about context references can prevent memory leaks, especially with components like Activities and Contexts.
Singletons, when used judiciously, can be a powerful pattern in Android development for efficient resource management and providing a cohesive mechanism for operation coordination.
Q25. How do you implement push notifications in Android?
Implementing push notifications in Android typically involves using Firebase Cloud Messaging (FCM), Google’s cross-platform messaging solution that enables you to send messages and notifications for free.
The process can be summarized in the following steps:
- Setup Firebase in Android Project: Add Firebase to your Android project by using the Firebase console. This involves adding a google-services.json file to your project and including Firebase dependencies in your “build.gradle” files.
- Create a Firebase Messaging Service: Extend “FirebaseMessagingService” and override onMessageReceived for handling messages and onNewToken for handling token generation. This service will be responsible for receiving messages from FCM.
- Register the Service in the Manifest: Declare your FirebaseMessagingService in the AndroidManifest.xml to ensure it’s recognized by the system.
- Sending Notifications: Use the Firebase console, your server, or another backend service to send notifications to your app’s users. When a message is received, use the Notification Manager in Android to build and display the notification.
- Handle Notification Clicks: Define intents and specify pending intents for your notifications to handle user clicks and navigate the user to parts of your app.
Implementing push notifications with FCM not only allows for efficient message delivery but also provides a broad set of features like targeting messages by user segment, scheduling messages, and tracking message analytics.
Q26. What is “Room”, and how does it facilitate database access in Android?
“Room” is part of the Android Architecture Components, serving as an abstraction layer over SQLite to provide a more robust database access mechanism while retaining the full power of SQLite. “Room” simplifies database work by providing compile-time checks of SQLite queries and reducing boilerplate code needed to connect to the database.
Key features of Room include:
- Compile-time Verification: “Room” validates SQL queries at compile time, catching errors before they result in runtime exceptions.
- Easy Database Migration: “Room” provides support for database migrations, helping developers handle schema changes smoothly.
- Integration with “LiveData” and “RxJava“: “Room” can return query results as “LiveData” or “Flowable” objects, automatically running the query asynchronously and providing observable data that can react to database changes.
To use Room, we:
- Define an Entity to represent a table in your database.
- Create a DAO (Data Access Object) with methods to access your database.
- Declare a Database class that is annotated with “@Database“, listing your entities and DAOs.
Room manages the creation and querying of the database, significantly reducing the complexity and code required to interact with SQLite databases in Android apps.
Q27. Explain the concept of data binding in Android.
Data Binding in Android is a support library feature that allows you to bind UI components in your layouts to data sources in your app using a declarative format rather than programmatically. It reduces boilerplate code, making your codebase cleaner and more readable.
Key aspects of data binding include:
- Layout and Data Integration: By adding a “<layout>” tag as the root element of your XML layout files and defining variables within “<data>” elements, you can directly link your UI components to data models.
- Automatic UI Updates: When data changes, the bound UI components are automatically updated without the need for manual intervention, improving efficiency in UI management.
- Event Handling: Data binding can also simplify event handling by binding layout components to methods in your activity or view model.
To enable data binding in your project, you must add the dataBinding element to your build.gradle file.
Then, in your layout XML, wrap your view hierarchy with a “<layout“> tag and define data variables.
Data binding streamlines the process of creating interactive layouts by allowing you to bind UI components directly to data sources, reducing the need for UI-related boilerplate code in your activities or fragments.
Q28. How do you manage app state and handle navigation in Android?
In Android, managing app state and handling navigation effectively involves using a combination of components and libraries designed to facilitate these tasks:
- ViewModel: To manage the UI-related data in a lifecycle-conscious way, ensuring data survives configuration changes such as screen rotations. ViewModel stores the data needed for the UI and separates it from the View logic.
- SavedInstanceState: For managing the app state across system-initiated process death, use the SavedInstanceState bundle to save and restore the UI state. This is critical for handling small amounts of UI data when the system kills the app for resource management.
- Jetpack Navigation Component: For handling app navigation, the Navigation component provides a framework for navigating between ‘destinations’ within an Android application. It simplifies implementing navigation, such as replacing fragments, handling Up and Back actions correctly by default, and providing consistent animation and transition behaviors.
- “NavHostFragment“: Acts as a container for destinations.
- “NavController“: Manages app navigation within a NavHost.
- “NavGraph“: Defines all navigation paths within the app.
- “LiveData“ or “StateFlow“: To observe data changes and update the UI accordingly, LiveData (for Java) or StateFlow (for Kotlin) can be used. These components are lifecycle-aware and ensure the UI corresponds to the current state of the app’s data.
- “DataStore“ or “SharedPreferences“: For persisting key-value pairs or small sets of data across app restarts, which can be part of the app’s state management strategy.
Q29. What is the difference between “ConstraintLayout” and “LinearLayout”?
“ConstraintLayout” and “LinearLayout” are both “ViewGroup” subclasses used to arrange child views on the screen, but they differ significantly in how they do so and the flexibility they offer:
- “LinearLayout“: Arranges child views in a single direction, either vertically or horizontally. It is straightforward to use but can lead to nested layouts (a layout inside another layout), which can degrade performance due to increased complexity in measuring and laying out views.
- “ConstraintLayout“: A more flexible and complex layout manager that allows you to position and size widgets based on constraints to other widgets in the layout and to the parent layout itself. It supports flat layout hierarchies (reducing the need for nested layouts), which improves performance. “ConstraintLayout” enables more complex layouts with relative positioning without the need for nesting, making it more efficient and versatile for designing UIs.
Overall, while “LinearLayout” is simpler and may be more intuitive for straightforward layouts, “ConstraintLayout” offers greater flexibility and efficiency, especially for more complex designs, by minimizing the need for nested view groups and providing more precise control over the positioning and sizing of child views.
Q30. How do you optimize “RecyclerView” performance in Android?
Optimizing “RecyclerView” performance involves several key practices:
- “ViewHolder“ Pattern: Ensure you’re using the “ViewHolder” pattern correctly to minimize the number of calls to “findViewById“, reducing costly layout inflation and view lookup operations. RecyclerView naturally encourages this pattern.
- Efficient Layouts: Use simple and flat layouts for list items. Avoid nested layouts as much as possible, as they increase the complexity of layout passes, impacting performance.
- Recycle Views: Make sure you’re recycling views properly by leveraging the adapter’s onBindViewHolder method to reuse view holders for different data items, minimizing unnecessary inflations.
- Load Images Efficiently: When displaying images, use image loading libraries like “Glide” or “Picasso” that handle loading and caching efficiently, reducing memory usage and avoiding UI thread blockage.
- Data Handling: Update your adapter’s data efficiently by using appropriate methods like “notifyItemChanged(int)“, “notifyItemInserted(int)“, etc., instead of “notifyDataSetChanged()” to minimize refresh operations and enable animations.
- Item Decorations and Animations: Use item decorations sparingly and opt for simple animations. Complex decorations and animations can slow down scrolling performance.
- Prefetching: Enable prefetching in “RecyclerView” to load data before it’s needed during scrolling, making scroll movements smoother.
- Use “DiffUtil“: For data updates, use “DiffUtil” to calculate the difference between old and new data sets and update only what’s necessary, improving update efficiency.
Q31. Discuss error-handling strategies in Android applications.
Effective error handling in Android applications involves anticipating potential issues, gracefully managing exceptions, and ensuring a positive user experience, even when errors occur. Key strategies include:
- “Try-Catch“ Blocks: Use “try-catch” blocks judiciously to catch and handle exceptions. Avoid generic catch-all exceptions; instead, catch specific exceptions to handle known issues precisely.
- Input Validation: Validate user inputs and data fetched from networks or databases to ensure they meet the expected format and criteria before processing, reducing the chance of errors.
- Null Checks: Perform null checks or use Kotlin’s null safety features to prevent null pointer exceptions, one of the most common crash causes in Android apps.
- Use of “LiveData“ and “Kotlin Flow“: Utilize “LiveData” or “Kotlin Flow” for managing data that automatically handles lifecycle states, reducing the risk of accessing views in inappropriate states.
- Error Reporting: Implement error reporting tools like “Firebase Crashlytics” to capture unhandled exceptions and crashes. These tools help identify and diagnose issues that occur in production.
- User Feedback: Provide clear, user-friendly error messages or feedback when errors occur, ensuring users understand what happened and possibly how to resolve it.
- Fail Gracefully: Design your app to fail gracefully, ensuring that a failure in one part doesn’t completely crash the app. Provide alternatives or recovery options when possible.
- Testing: Employ thorough testing strategies, including unit tests, integration tests, and UI tests, to catch and fix errors early in the development cycle.
Q32. What are Annotations in Java/Kotlin, and how are they used in Android?
In Java, annotations are defined before class, method, or variable declarations using the “@” symbol, such as “@Override“, “@NonNull“, or custom annotations. Kotlin uses a similar syntax, also allowing annotations on constructors, properties, type parameters, and more.
Key uses of annotations in Android include:
- Compile-time Checks: Annotations like “@NonNull” and “@Nullable” inform the compiler about nullability constraints, improving code safety by catching potential null pointer exceptions at compile time.
- Dependency Injection: Frameworks like Dagger use annotations (“@Inject“, “@Module“, “@Component“) to identify dependency injection points, reducing the need for manual boilerplate dependency injection code.
- Data Persistence: Room uses annotations (“@Entity“, “@Dao“, “@Insert“, “@Delete“) to map classes to database tables and define data access objects, streamlining database operations.
- Serialization/Deserialization: Libraries like “Gson” use annotations (“@SerializedName“) to define how objects are converted to JSON and vice versa, simplifying data parsing.
- Testing: Annotations (“@Test“, “@Before“, “@After“) identify methods as test cases or setup/teardown methods in testing frameworks like JUnit.
Q33. How do you secure sensitive data in Android applications?
Securing sensitive data in Android applications involves implementing a multi-layered approach to protect data both at rest and in transit. Key strategies include:
- Encryption: Use encryption for data stored on the device at rest. Android provides APIs like “EncryptedSharedPreferences” and the Android “Keystore” system for encrypting data and securely storing encryption keys.
- Secure Transmission: Ensure all data transmitted over the network is done over secure protocols such as HTTPS, using TLS/SSL encryption to protect data in transit.
- Data Minimization: Only collect and store the minimum amount of sensitive data necessary for your app to function. Often, the best way to secure data is not to store it at all.
- Using “ProGuard“: Use “ProGuard” or “R8” for code obfuscation, which makes it harder for malicious users to analyze and reverse engineer your app, protecting against static analysis attacks.
- Secure Authentication: Implement strong authentication mechanisms, such as OAuth, and consider using biometric authentication for sensitive operations within your app.
- Regular Updates: Regularly update your app to address security vulnerabilities and ensure you are using the latest security best practices and APIs provided by Android.
- Access Control: Implement proper access control in your app, ensuring that sensitive information is only accessible to authenticated and authorized users.
- Third-Party Libraries: Be cautious when using third-party libraries; ensure they are reputable and regularly updated to avoid introducing security vulnerabilities into your app.
Q34. Explain the use of Broadcast Receivers in Android.
Broadcast Receivers in Android are components that enable applications to listen for and respond to broadcast messages from other applications or from the system itself. These messages, known as Intents, can be either explicitly targeted at a specific app or broadcasted to all apps that have registered to receive that particular action.
Key uses of Broadcast Receivers include:
- Listening for System Events: Such as boot completion, battery changes, connectivity changes, or screen off and on states. Apps can register to receive these system events and respond accordingly, for example, starting a service once the device has been booted.
- Inter-App Communication: Broadcast Receivers allow apps to communicate with each other without direct interaction. An app can send a broadcast to inform other apps of specific events, like a new photo being saved to the gallery.
- Scheduling Repeating Events: Together with “AlarmManager“, Broadcast Receivers can be used to schedule recurring tasks in a power-efficient manner, even if the app itself isn’t running.
Broadcast Receivers can be declared in the app’s manifest file for system-wide broadcasts or registered dynamically in the code for more contextual events. It’s important to manage receivers properly, unregistering them when not needed to conserve system resources.
Other Tech Interview Questions Lists
- Java Interview Questions
- Python Interview Questions
- JavaScript Interview Questions
- iOS Interview Questions
- NoSQL DB Interview Questions for Freshers
- NoSQL Interview Questions for Experienced
- Data Engineer Interview Questions
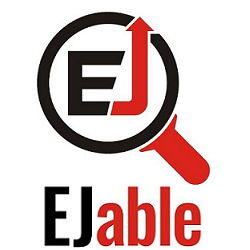
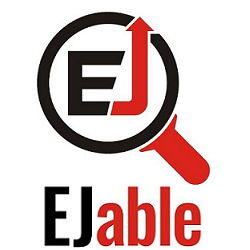